Go Channels Demystified: Practical Use Cases and Best Practices
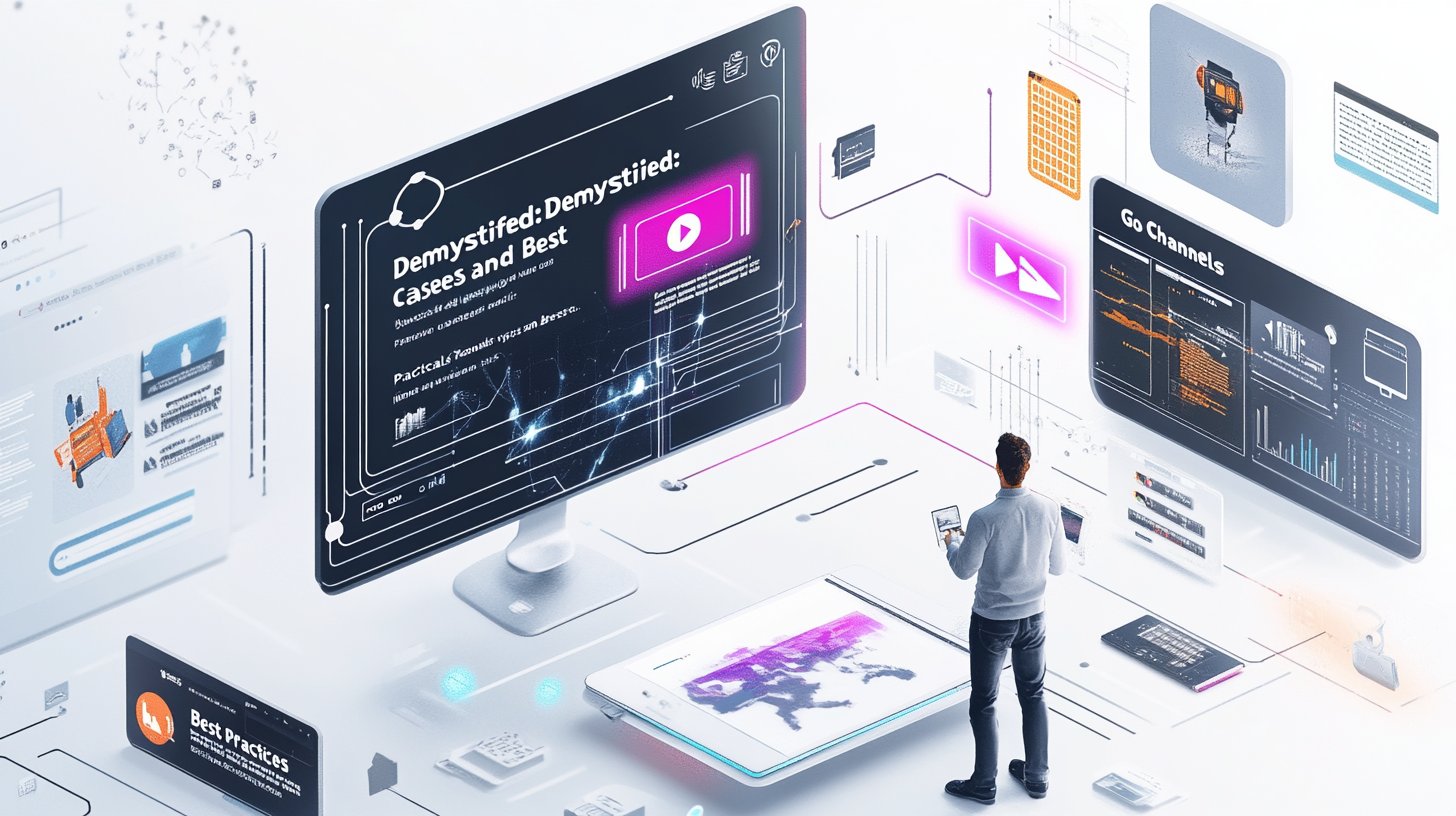
Go channels are a powerful feature of the Go programming language that facilitate communication and synchronization between goroutines. They are a fundamental building block for concurrent programming in Go, allowing developers to create efficient and scalable applications. This article will demystify Go channels, explore their practical use cases, and provide best practices for working with them effectively.
1. Understanding Go Channels
What are channels?
Channels in Go are typed conduits through which you can send and receive values. They act as a communication mechanism between goroutines, allowing them to synchronize execution and exchange data without explicit locks or condition variables.
Types of channels
Go supports two types of channels:
- Unbuffered channels: These channels have no capacity and require both the sender and receiver to be ready at the same time for communication to take place.
- Buffered channels: These channels have a capacity and can hold a specified number of values before the sender blocks.
Channel operations
The three primary operations on channels are:
- Send:
ch <- v
- Receive:
v := <-ch
- Close:
close(ch)
Here’s a simple example demonstrating channel usage:
func main() {
ch := make(chan int)
go func() {
ch <- 42
}()
value := <-ch
fmt.Println(value) // Output: 42
}
2. Practical Use Cases
Producer-Consumer pattern
The producer-consumer pattern is a classic use case for channels. It involves one or more producer goroutines generating data and one or more consumer goroutines processing that data.
Example:
func producer(ch chan<- int) {
for i := 0; i < 10; i++ {
ch <- i
}
close(ch)
}
func consumer(ch <-chan int) {
for num := range ch {
fmt.Println("Consumed:", num)
}
}
func main() {
ch := make(chan int)
go producer(ch)
consumer(ch)
}
Fan-out, Fan-in
Fan-out is the process of distributing work across multiple goroutines, while fan-in is the process of combining the results from multiple goroutines into a single channel.
Example:
func fanOut(in <-chan int, n int) []<-chan int {
channels := make([]<-chan int, n)
for i := 0; i < n; i++ {
ch := make(chan int)
go func() {
for num := range in {
ch <- num * 2
}
close(ch)
}()
channels[i] = ch
}
return channels
}
func fanIn(channels ...<-chan int) <-chan int {
out := make(chan int)
var wg sync.WaitGroup
wg.Add(len(channels))
for _, ch := range channels {
go func(c <-chan int) {
for num := range c {
out <- num
}
wg.Done()
}(ch)
}
go func() {
wg.Wait()
close(out)
}()
return out
}
func main() {
in := make(chan int)
go func() {
for i := 1; i <= 10; i++ {
in <- i
}
close(in)
}()
channels := fanOut(in, 3)
out := fanIn(channels...)
for result := range out {
fmt.Println(result)
}
}
Timeouts and cancellation
Channels can be used in combination with the select
statement to implement timeouts and cancellation mechanisms.
Example:
func worker(ctx context.Context) <-chan int {
ch := make(chan int)
go func() {
defer close(ch)
for {
select {
case <-ctx.Done():
return
case <-time.After(time.Second):
ch <- rand.Intn(100)
}
}
}()
return ch
}
func main() {
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel()
ch := worker(ctx)
for num := range ch {
fmt.Println("Received:", num)
}
fmt.Println("Worker stopped")
}
Worker pools
Channels are excellent for implementing worker pools, where a fixed number of goroutines process tasks from a shared queue.
Example:
func worker(id int, jobs <-chan int, results chan<- int) {
for job := range jobs {
fmt.Printf("Worker %d processing job %d\n", id, job)
time.Sleep(time.Second)
results <- job * 2
}
}
func main() {
jobs := make(chan int, 100)
results := make(chan int, 100)
for w := 1; w <= 3; w++ {
go worker(w, jobs, results)
}
for j := 1; j <= 9; j++ {
jobs <- j
}
close(jobs)
for a := 1; a <= 9; a++ {
<-results
}
}
Pipelines
Channels can be used to create pipelines, where the output of one stage becomes the input of the next stage.
Example:
func generator(nums ...int) <-chan int {
out := make(chan int)
go func() {
for _, n := range nums {
out <- n
}
close(out)
}()
return out
}
func square(in <-chan int) <-chan int {
out := make(chan int)
go func() {
for n := range in {
out <- n * n
}
close(out)
}()
return out
}
func main() {
c := generator(1, 2, 3, 4)
out := square(c)
for n := range out {
fmt.Println(n)
}
}
3. Best Practices
Proper channel sizing
- Use unbuffered channels for synchronization between goroutines.
- Use buffered channels when you know the number of values that will be sent in advance.
- Avoid using very large buffer sizes, as they can hide synchronization issues.
Avoiding deadlocks
- Ensure that for every send operation, there’s a corresponding receive operation.
- Use
select
statements with default cases to prevent blocking indefinitely. - Implement timeouts to break potential deadlocks.
Closing channels
- The sender should close the channel when no more values will be sent.
- Receivers can check if a channel is closed using the comma-ok idiom:
v, ok := <-ch
- Never close a channel from the receiver side.
Error handling with channels
- Use a separate error channel or a struct that includes an error field.
- Implement graceful error handling and propagation in channel-based workflows.
Example:
type Result struct {
Value int
Err error
}
func worker(jobs <-chan int, results chan<- Result) {
for job := range jobs {
if job%2 == 0 {
results <- Result{Value: job * 2, Err: nil}
} else {
results <- Result{Value: 0, Err: fmt.Errorf("odd number: %d", job)}
}
}
}
func main() {
jobs := make(chan int, 5)
results := make(chan Result, 5)
go worker(jobs, results)
for i := 1; i <= 5; i++ {
jobs <- i
}
close(jobs)
for i := 1; i <= 5; i++ {
result := <-results
if result.Err != nil {
fmt.Println("Error:", result.Err)
} else {
fmt.Println("Result:", result.Value)
}
}
}
Testing channel-based code
- Use buffered channels in tests to prevent blocking.
- Implement timeouts in tests to catch deadlocks.
- Use the
sync/errgroup
package for managing multiple goroutines in tests.
4. Advanced Patterns
Select statement
The select
statement allows you to wait on multiple channel operations simultaneously.
Example:
func main() {
ch1 := make(chan string)
ch2 := make(chan string)
go func() {
time.Sleep(2 * time.Second)
ch1 <- "one"
}()
go func() {
time.Sleep(1 * time.Second)
ch2 <- "two"
}()
for i := 0; i < 2; i++ {
select {
case msg1 := <-ch1:
fmt.Println("Received", msg1)
case msg2 := <-ch2:
fmt.Println("Received", msg2)
}
}
}
Buffered vs. Unbuffered channels
Understand the differences and use cases for buffered and unbuffered channels:
- Unbuffered channels: Synchronous communication, sender blocks until receiver is ready.
- Buffered channels: Asynchronous communication up to the buffer size, sender only blocks when buffer is full.
Directional channels
Use directional channels to clarify the intent of your functions and prevent misuse.
Example:
func ping(pings chan<- string, msg string) {
pings <- msg
}
func pong(pings <-chan string, pongs chan<- string) {
msg := <-pings
pongs <- msg
}
func main() {
pings := make(chan string, 1)
pongs := make(chan string, 1)
ping(pings, "passed message")
pong(pings, pongs)
fmt.Println(<-pongs)
}
5. Common Pitfalls and How to Avoid Them
Sending on a closed channel: This will cause a panic. Always ensure the channel is open before sending.
Forgetting to close channels: This can lead to goroutine leaks. Close channels when no more values will be sent.
Closing channels multiple times: This will cause a panic. Implement a mechanism to ensure a channel is closed only once.
Deadlocks due to incorrect channel usage: Ensure proper synchronization between senders and receivers.
Race conditions: Use the
-race
flag when testing and thesync/atomic
package for concurrent access to shared variables.
Go channels are a powerful tool for building concurrent applications. By understanding their behavior, use cases, and best practices, you can leverage channels to create efficient, scalable, and maintainable Go programs. Remember to always consider the synchronization requirements of your application and choose the appropriate channel type and pattern for each situation.
As you continue to work with channels, experiment with different patterns and continuously refine your understanding of Go’s concurrency model. With practice, you’ll be able to write robust concurrent programs that take full advantage of Go’s capabilities.